Docker Basics
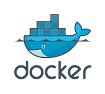
Exercise 1: Hello world
Suppose that we have a bash script named helloworld.sh that prints "$NAME: Hello World!".
-
Build a docker image
- based on Ubuntu 18.04.
- Set the working directory to /usr/src/app
- Copy helloworld.sh to the working directory
- Set environment variable NAME to your name.
- Make the script executable using chmod command.
- Run the script when the container starts.
- List all images.
-
Run a container with name hw, with your image.
- List all containers.
- Stop and remove the container.
Jump to solution
Exercise 2: Keep container running
- based on Ubuntu 18.04.
- Set the working directory to /usr/src/app
- Copy helloworld.sh to the working directory
- Set environment variable NAME to your name.
- Make the script executable using chmod command.
- Run the script when the container starts.
-
Run a container with name hw with your image, and detach it
and keep it running.
- Bash to the container and run the helloworld script.
-
Stop and remove the hw container.
Jump to solution
Exercise 3: Mount a folder
- Create a folder named
- Run the container again and mount the folder to it.
- Inspect the container to make sure the folder has been mounted.
- Bash into the container and put a file in the mounted folder.
- Check the file in the host.
- Stop and remove the hw container.
Jump to solution
Exercise 4: Deploy a hello-world server
- Write a nodejs webserver that listens on port 3000, and responds "Hello World"
- Create an image that runs this webserver upon running.
-
Run a container with name hw, with your image in the background.
- Go to http://localhost:3000, you must see "Hello World".
-
Stop and remove hw container.
Jump to solution
Exercise 5: Push your image to a registry
- Login to your dockerhub account via docker
- Push your local helloworld image to your dockerhub repository.
- Remove your helloworld image.
Solutions
We first create a Dockerfile in the folder that we have our
HelloWorld.sh script.
Now, we run the following command in the same folder:
docker build --tag helloworld .
To list all images:
docker image ls
To run a container out of this image:
docker run --name hw helloworld
To list containers:
docker container ls
To stop the container:
docker stop hw
To remove the container:
docker rm hw
First, comment out the CMD line in the Dockerfile, and create a new image.
To run the container in background and keep it running:
docker run
To bash to the container:
docker exec -it hw bash
Exercise 3
To mount the folder: docker run
docker run
To inspect:
docker inspect hw
and check "Mounts".
Exercise 4
Nodejs webserver:
Note that, you should not specify hostname as localhost, as this way you cannot access the webserver from the host machine.
First, we create a Dockefile from node official image. We name it helloworld_server.
To run the image and make port 3000 accessible for it:
docker run --publish 3000:3000 --detach --name hws helloworld_server
Now, http://localhost:3000 must show "Hello World".
Now, first, we tag our existing image:
docker tag helloworld myusername/myrepo:new_tag
Note that the new_tag is optional.
To push:
docker push myusername/myrepo:new_tag
To remove the image:
docker image rm helloworld
Comments
Post a Comment